클래스 상속이란? -> 부모 클래스의 행위, 속성 상속을 의미한다.
옵젝씨는 단일 상속을 지원함!
iOS 개발에서 만나는 상속
- 뷰(부모 클래스)와 레이블, 이미지 뷰, 버튼
- 뷰의 속성과 행위(위치, 크기, 화면 표시)를 상속
- 상속을 통해서 상세화, 구체화됨
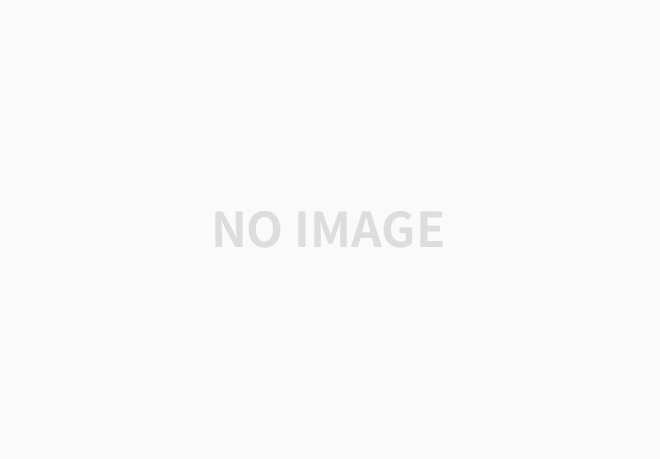
1. 자식 클래스 생성
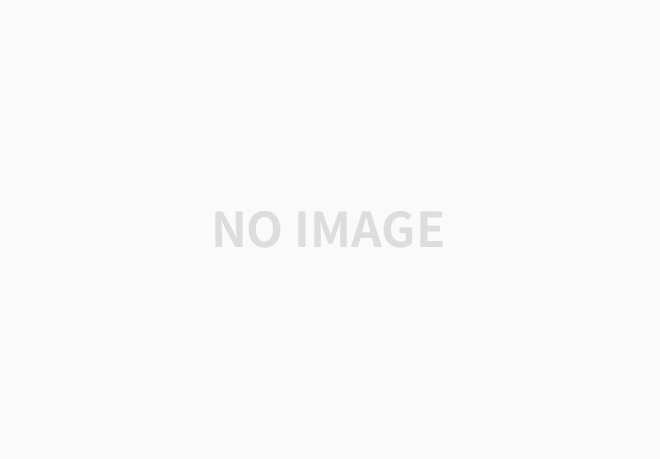
Subclass of -> 부모 클래스를 의미함
#import "Rectangle.h"
@interface Square : Rectangle
@end
2. 상속과 is-a 관계
자식 클래스 객체는 부모 클래스 객체로 취급
- 뷰 관계에서의 is-a 관계
UIView *view1 = [[UILabel alloc]init];
UIView *view2 = [[UIImageView alloc]init];
- 사각형과 정사각형에서의 is-a 관계
- Square는 Rectangle 이다.
Rectangle *obj = [[Square alloc]init];
3. 메소드 재정의
오버라이딩(overriding)
상속: 부모 클래스의 메소드 상속
메소드 재정의: 부모 클래스의 메소드를 재정의
예제)
1. 정사각형의 가로 길이 설정을 재정의
2. 가로와 세로 길이 동시 설정
#import "Square.h"
@implementation Square
-(void)setWidth:(int)newWidth{
width = newWidth;
height = newWidth;
}
@end
4. 오버로딩
오버로딩 : 같은 메소드를 파라미터를 구분
다만, 오브젝티브 씨에서는 오버로딩을 지원 안함.
-(void)foo:(int)i;
-(void)foo:(NSString *)str;
따라서 레이블을 다르게 작성 해줘야 한다.
-(void)foo:(int)a with:(float)b;
-(void)foo:(int)a second:(float)b;
5. 포인터 super
self : 클래스 내부에서 동작 둥인 객체 참조
super : 부모 클래스 부분을 참조
Square에서 부모 클래스 Rectangle 기능 사용
-(void)setWidth:(int)newWidth {
[super setWidth:newWidth];
[super setHeight:newHeight];
}
'IOS > Objective-C' 카테고리의 다른 글
[Objective-C] 객체 생성 메소드 (0) | 2023.03.09 |
---|---|
[Objective-C] 동적 타입과 바인딩 (0) | 2023.03.09 |
[Objective-C] Class 만들어보기 (0) | 2023.02.28 |
[Objective-C] 기본 표기법 및 객체 지향 프로그래밍 (0) | 2023.02.27 |
[Objective-C] Objective-C 파일 살펴보기 (0) | 2023.02.26 |